Loop Control Statements in C
Introduction to HTML
How to use the Github API
The image tag, anchor tag and the button tag
Ordered and Unordered Lists in HTML
The division tag
HTML Forms
Tables in HTML
Introduction to C Programming
Introduction to Python
Varibles and Datatypes in Python
Operators in Python
Typecasting in Python
Input and Output in Python
If Else in Python
Loops in Python
Break, Continue and Pass in Python
Python practice section 1
Lists in Python
Tuple in Python
Varibles and Datatypes in Python
Variable
Variable is a container that stores data. For example, we have a bottle it stores water. Here bottle is
variable and water is data. As we know everything in our system is stored in memory. So we can say, a
variable is a name given to the memory location in which we store data.
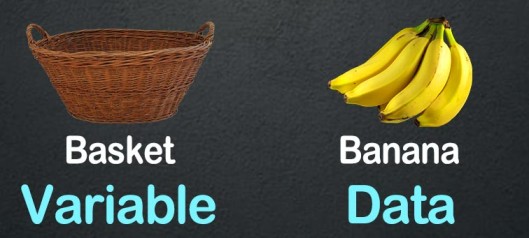
Example of Variable:-
n = 100
m = 10.43
s = "Rapid Coders"
Here n, m, s are variables.
Rules for defining the name of variable:
- Variable name can contain alphabets, digits, and underscore
- Variable name can not be any keyword. Keywords are words that have special meanings.
- Variable name should not contain any special character.
- Variable name can only start with alphabet or underscore and can not start with digit
- White spaces are not allowed in variable
Example of valid variable names:- x, y, abc, username, etc.
Note:- Always use meaningfull name for variables. As we know spaces are not allowed so
we use the following naming conventions:
- Camel case:- In this convention first letter of the variable is lowercase and all other words have the first letter in uppercase. Example:- nameOfUser, ageOfPerson.
- Snake case:- In this convention, we use underscore where we need to use space. Example:- name_of_user, age_of_person.
Datatypes
Type of data is called datatype. Before understanding it technically let's understand it generally. In
real life, we have apples, bananas, mango, etc. These all are fruits. Here apples and bananas are data
and fruit is datatype. Technically datatype is a classification that specifies the type of value or
data.
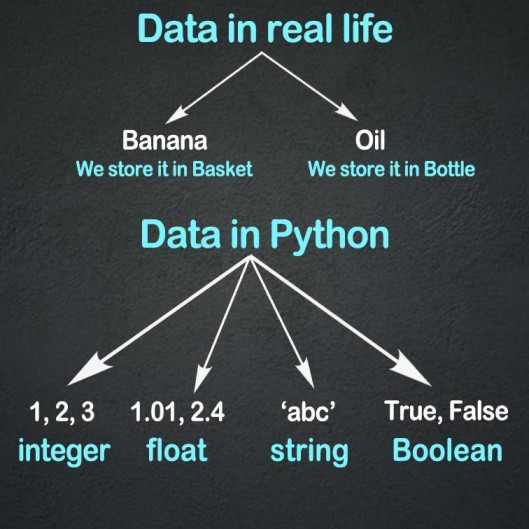
In Python we have the following datatypes:
- Integers:- An integer is a whole number and it can be positive, negative, or zero. Example: 1, 2, 43, 456
- Floating point numbers:- A floating point number, is a positive or negative number with a decimal point. Example: 1.34, 2.543, 43.01, 456.5
- String:- String is a collection of characters. Example: "rapid coders", "Akshay"
- Booleans:- They can have only two values which are True and False.
- None:- This mean nothing. It is used to represent null or no value.
Some other datatypes are:
- List
- Tuple
- set
- dictionary
We will learn about them in further articles.