Loop Control Statements in C
Introduction to HTML
How to use the Github API
The image tag, anchor tag and the button tag
Ordered and Unordered Lists in HTML
The division tag
HTML Forms
Tables in HTML
Introduction to C Programming
Introduction to Python
Varibles and Datatypes in Python
Operators in Python
Typecasting in Python
Input and Output in Python
If Else in Python
Loops in Python
Break, Continue and Pass in Python
Python practice section 1
Lists in Python
Tuple in Python
Typecasting in Python
Typecasting means converting data from one type to another. In real life, we see many examples
of typecasting such as converting fruit to juice or electricity to light, and much more. In all
examples, data of one type is converted into another as per the requirement.
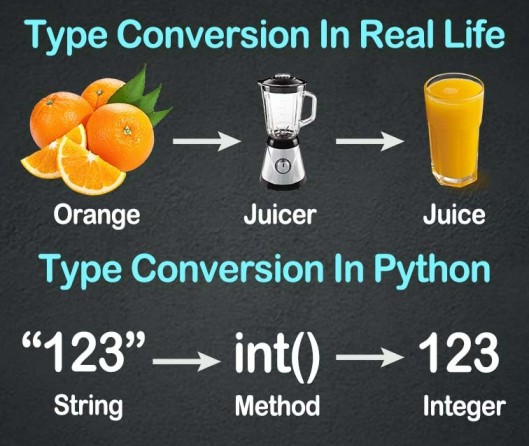
We have two types of typecasting :
1. Implicit Type Conversion:- When a variable or data of one type mix with variable or data of
another type, type conversion or typecasting takes place. It is done automatically. For example -
a = 5
print(type(a))
b = 2.3
print(type(b))
d = a*b
print(d)
print(type(d))
Output
<class 'int'> <class 'float'> 11.5 <class 'float'>
When the program is run, the variable 'a' is automatically converted into float, this happens because
the operator performs an operation on the same type of operants.
2. Explicit Type Conversion:- It is done by the user. We use explicit type casting when we want
to specify the data type of a variable, or we want to change the data type of a variable. We use
constructor functions to do explicit type conversion. Functions used in typecasting are:
- int():- It is used to convert float to int or string to int.
- float():- It is used to convert int to float or string to float.
- str():- It is used to convert int to string or float to string.
Examples of Explicit type casting:-
Conversion from float to int
a = 6.21
print(type(a)) # type() function is used to get type of object
b = int(a) # converts float into int
print(b)
print(type(b))
Output
<class 'float'> 6 <class 'int'>
Conversion from string to integer
a = "1234"
print(type(a)) # type() function is used to get type of object
b = int(a) # converts string into int
print(b)
print(type(b))
Output
<class 'str'> 1234 <class 'int'>