Introduction to HTML
The image tag, anchor tag and the button tag
The division tag
Ordered and Unordered Lists in HTML
Tables in HTML
HTML Forms
HTML Forms
Forms are commonly used to gather specific information from a group of people.
It includes entries such as name, phone number, age etc where users can fill in their
personal info. In HTML, we can create a form using the <form> tag.
- The <input> tag
- The <label> tag
- The <select> tag
- Final form
Jump to specific sections
The <input> tag
It is used to take input from the user. This tag has an attribute type which defines the type
of input it can take. Input is of various types such as text, date, numeric etc. We can also take
passwords as input. When the user will enter the password, it will show up as circles without revealing.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
</head>
<body>
<form>
<div> Username
<input type="text">
</div>
<br>
<div> Password
<input type="password">
</div>
<br>
<div> Age
<input type="number">
</div>
<br>
<div> Enter your date of birth
<input type="date">
</div>
<br>
<div> All the information provided is true.
<input type="checkbox">
</div>
</form>
</body>
</html>
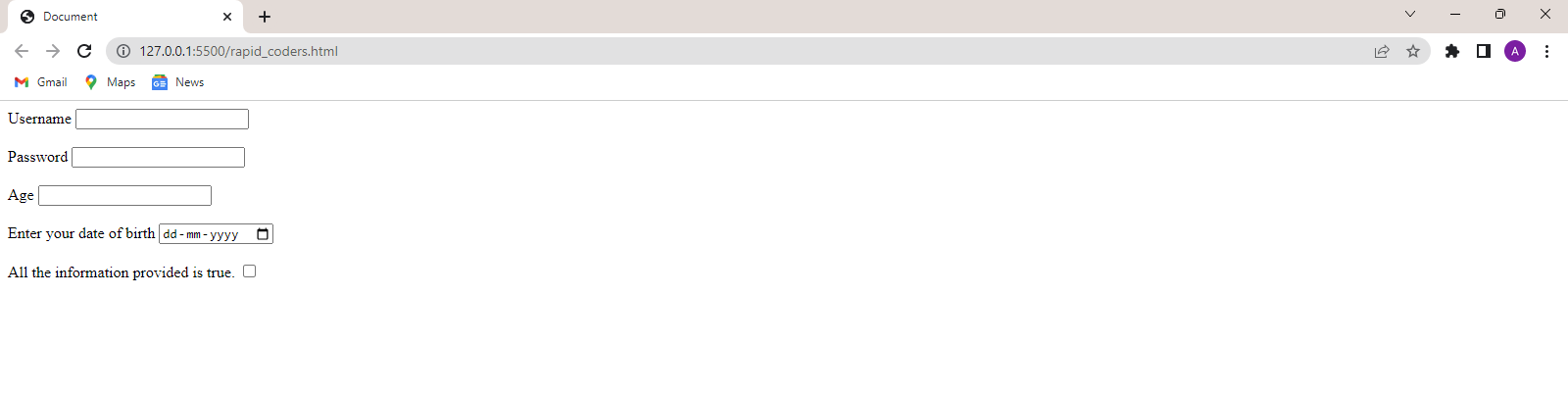
The <input> tag has a display inline. So,we have used the <div> tag for better layout.
There are many more input types. To check all of them, check out this post.
The <label> tag
The <label>tag is used to define the heading of a input field like username,password etc. It helps to select the associated input field.The for attribute must contain
the id of the associated input field. The id attrihbute is used to uniquely identify
a tag in a HTML document. It is used as follows:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>HTML Forms</title>
</head>
<body>
<form action="">
<div>
<label for="username">Username</label>
<input type="text" id="username" name="username">
</div>
<br>
<div>
<label for="password">Password</label>
<input type="password" id="password" name="password">
</div>
<button type="submit">Submit</button>
</form>
</body>
</html>

Our form is partially ready. The button with type="submit" submits the form
to the link specified in the action attribute of the <form> tag.
After submitting, the form resets to a new form. All the form fields can be accessed by using the name attribute after submission in backend.
The select tag
The <select> tag is used to give choices to the user. The user can select any one of them
by clicking on it. The <option> tag is used to specify the various choices. The value field
denotes the value of a particular option which can be accessed when the form gets submitted. It
can be used as follows.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<form action="">
<div>
<label for="name">Name</label>
<input type="text" id="name">
</div>
<br>
<div>
<label for="sport">Select your age group</label>
<select id="sport">
<option value="young">12-17</option>
<option value="adult">18-45</option>
<option value="old">45-above</option>
</select>
</div>
<br>
<button type="submit">Submit</button>
</form>
</body>
</html>

Final Form
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<form action="">
<div>
<label for="username">Username</label>
<input type="text" id="username" name="username">
</div>
<br>
<div>
<label for="password">Password</label>
<input type="password" id="password" name="password">
</div>
<br>
<div>
<label for="email">Email</label>
<input type="email" id="email" name="email">
</div>
<br>
<div>Choose a gender
<label for="male">Male</label>
<input type="radio" id="male" name="gender">
<label for="female">Female</label>
<input type="radio" id="female" name="gender">
</div>
<br>
<div>
<label for="age">Age</label>
<input type="number" id="age">
</div>
<br>
<div>
<label for="sport">Choose a sport</label>
<select id="sport">
<option value="Basketball">Basketball</option>
<option value="Volleyball">Volleyball</option>
<option value="Football">Football</option>
</select>
</div>
<br>
<button type="submit">Submit</button>
</form>
</body>
</html>
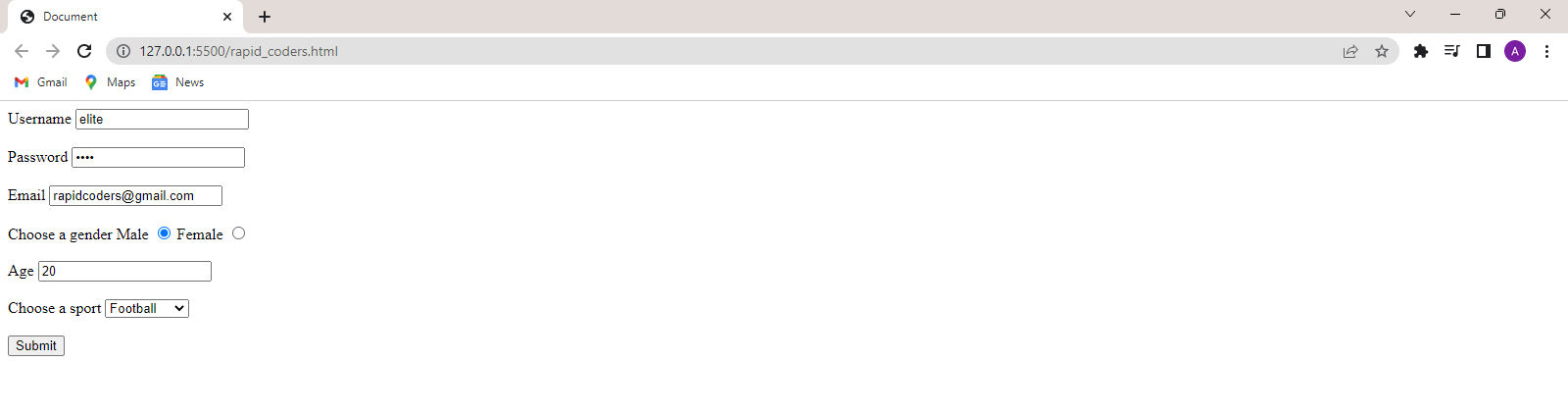