Loop Control Statements in C
Introduction to HTML
How to use the Github API
The image tag, anchor tag and the button tag
Ordered and Unordered Lists in HTML
The division tag
HTML Forms
Tables in HTML
Introduction to C Programming
Introduction to Python
Varibles and Datatypes in Python
Operators in Python
Typecasting in Python
Input and Output in Python
If Else in Python
Loops in Python
Break, Continue and Pass in Python
Python practice section 1
Lists in Python
Tuple in Python
How to use the Github API?
Github provides its API https://api.github.com/ to access a user's details like username, github avatar, followers, following, bio and details about repositories such as name, number of contributions and languages etc. Visit the official documentation page at
https://docs.github.com/en/rest?apiVersion=2022-11-28In this article,we are going to look at the various endpoints with the help of a Python Program.The no of accesses to the API are limited at 60 per hour. If you want to increase the no. of accesses,
you may generate an API Key from your Github Dashboard.In this article,we have used the API witout any
key.Now,
lets get started.
- Setting Up the API
- Access Specific fields
- Get the names of each followers.
- Get the names and description of all repositories.
- Get the no. of commits in a repository.
- Get all the languages used in a repository.
Jump to specific sections
Make your first request
The requests library is used to make GET requests to an endpoint.
import requests
request_url="https://api.github.com/users/Akshay2002Singh"
x=requests.get(request_url)
data=x.json()
print(data)
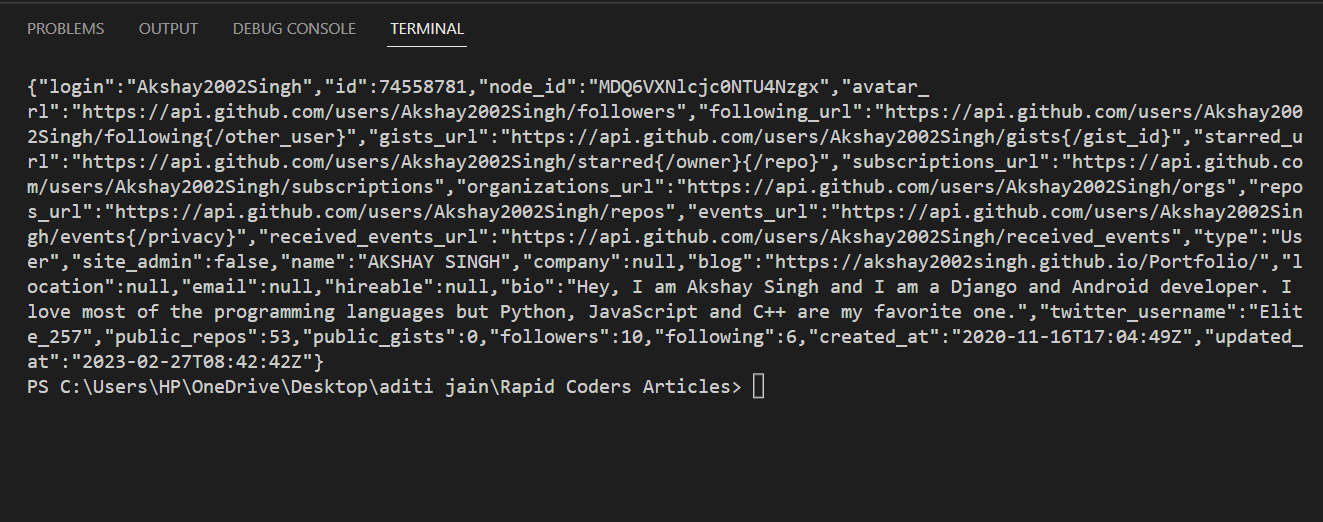
Here,we are having a request_string= https://api.github.com/users/[-Put github username
here-]".
Then,we made a request to the requested url using the requests.get() method to get the data.Next,
convert the data into json.
Access specific fields
To access the bio,we access the json object with key as "bio".We can also get the avatar link with the
key "avatar_url".
You can open the link in the browser to view or download the avatar image.
We can also get the date of creation of Github account using key "created_at".
## To get the bio
print("Bio is : ",data["bio"])
print()
print()
## To get the avatar link
print("Avatar link is : ",data["avatar_url"])
print()
print()
## To get the date of creation of Profile
print("Date of creation : ",data["created_at"])
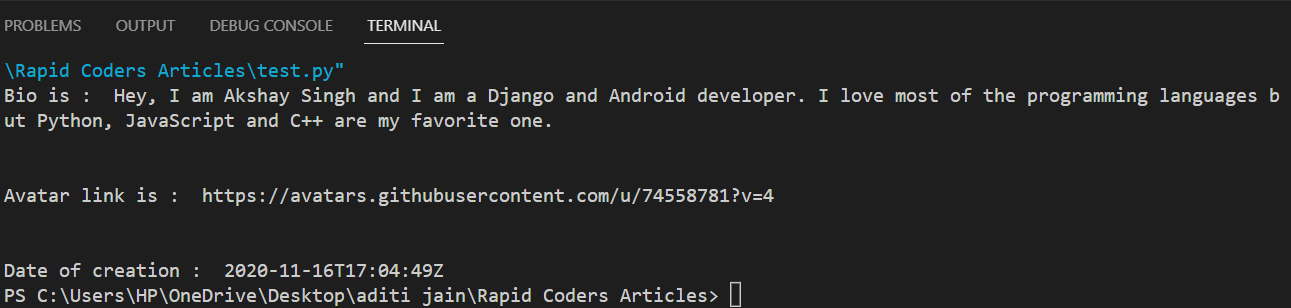
Get the names of all followers.
We can get a list of all followers by using the endpoint https://api.github.com/users/[-Put
github username here-]/followers.
This returns a json array of objects.We iterate through the array and print the name of each followers
follows.
# To get the names and profile links of followers
followers_url="https://api.github.com/users/Akshay2002Singh/followers"
f=requests.get(followers_url)
followers=f.json()
for follower in followers:
print(follower["login"])
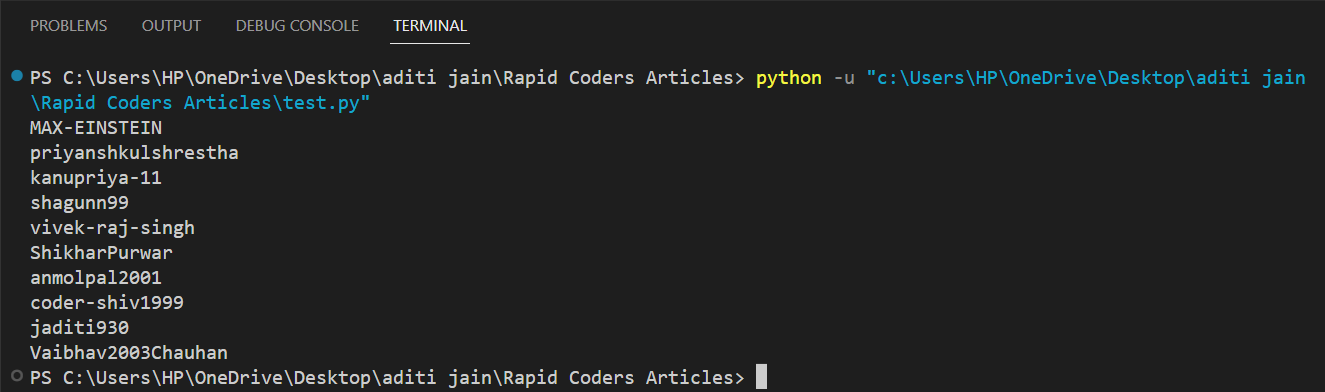
Get the names and description of all repositories.
To get this,we will use the endpoint https//api.github.com/users/[-Put github username
here-]/repos.This returns a
json array of objects.We iterate through the array and print the name of each repository along with
their descriptions.
## To get the name and description of repos
repos_url="https://api.github.com/users/Akshay2002Singh/repos"
r=requests.get(repos_url)
repos=r.json()
for repo in repos:
print("Repo : ",repo["name"])
print("Description : ",repo["description"])
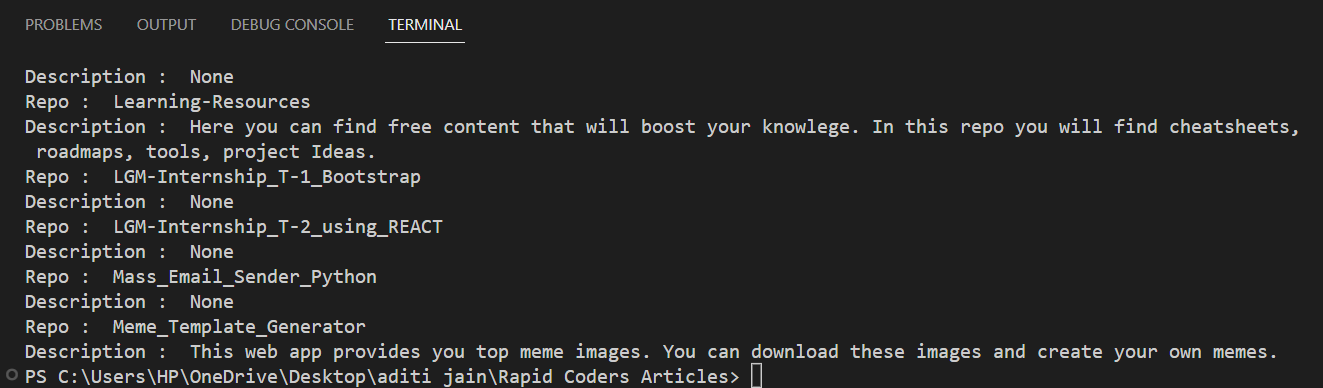
Get the no.of commits in a repository.
To get the no. of commits in the repository,we make a request to:
https://api.github.com/repos/Akshay2002Singh/Bootstrap_Website/contributorsThis again returns a json object.We can access the no.of commits by using the key "contributions".
commits_url="https://api.github.com/repos/Akshay2002Singh/Engineers-Library/contributors"
c=requests.get(commits_url)
commits=c.json()
print(commits[0]["contributions"])
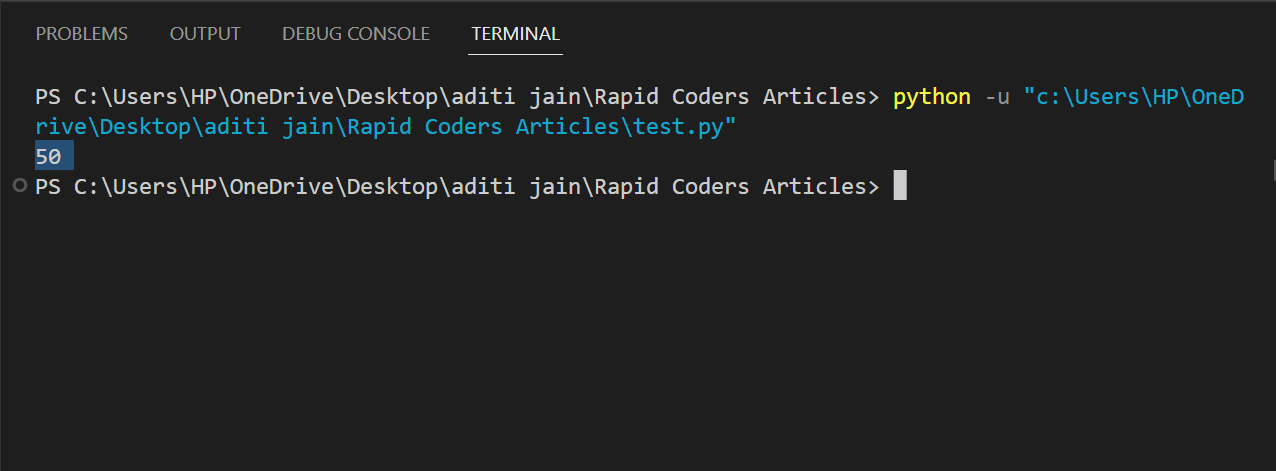
Get all the languages used in a repository.
To get the names of languages used,we make a request to the endpoint
https://api.github.com/repos/[-Put github username here-]/[-Repo Name goes
here-]/languages.
This return a json object containing all the languages.
lang_url="https://api.github.com/repos/Akshay2002Singh/Engineers-Library/languages"
l=requests.get(lang_url)
languages=l.json()
for language in languages:
print(language)
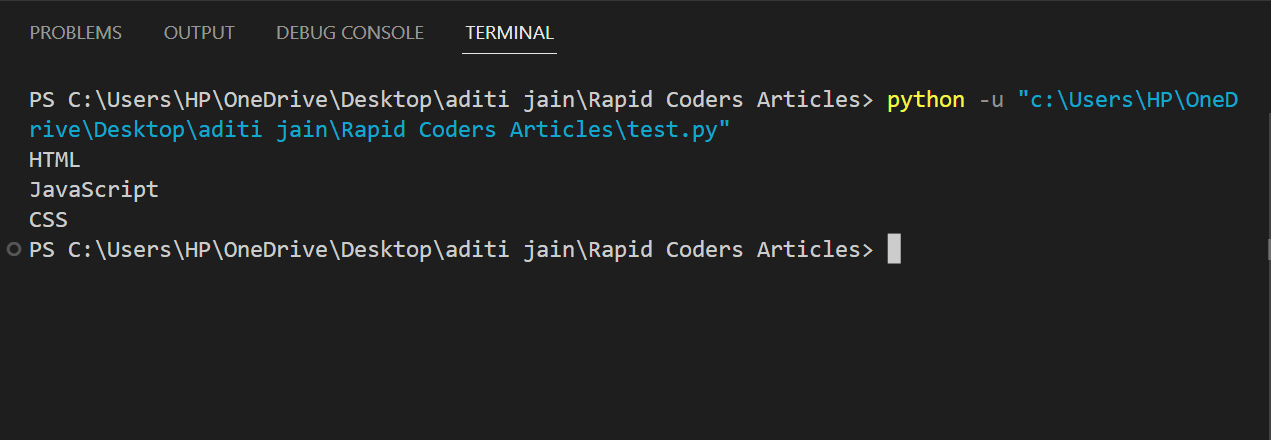
Final Code
import requests
request_url="https://api.github.com/users/Akshay2002Singh"
x=requests.get(request_url)
data=x.json()
# ## To get the bio
print("Bio is : ",data["bio"])
print()
print()
# ## To get the avatar link
print("Avatar link is : ",data["avatar_url"])
print()
print()
# ## To get the date of creation of Profile
print("Date of creation : ",data["created_at"])
# To get the names and profile links of followers
followers_url="https://api.github.com/users/Akshay2002Singh/followers"
f=requests.get(followers_url)
followers=f.json()
for follower in followers:
print(follower["login"])
## To get the name and description of repos
repos_url="https://api.github.com/users/Akshay2002Singh/repos"
r=requests.get(repos_url)
repos=r.json()
for repo in repos:
print("Repo : ",repo["name"])
print("Description : ",repo["description"])
## To get the no. of commits in a repository
commits_url="https://api.github.com/repos/Akshay2002Singh/Engineers-Library/contributors"
c=requests.get(commits_url)
commits=c.json()
print(commits[0]["contributions"])
## To get the languages in a repository
lang_url="https://api.github.com/repos/Akshay2002Singh/Engineers-Library/languages"
l=requests.get(lang_url)
languages=l.json()
for language in languages:
print(language)