Loop Control Statements in C
Introduction to HTML
How to use the Github API
The image tag, anchor tag and the button tag
Ordered and Unordered Lists in HTML
The division tag
HTML Forms
Tables in HTML
Introduction to C Programming
Introduction to Python
Varibles and Datatypes in Python
Operators in Python
Typecasting in Python
Input and Output in Python
If Else in Python
Loops in Python
Break, Continue and Pass in Python
Python practice section 1
Lists in Python
Tuple in Python
More about 2D arrays
In this article, we will learn about some core concepts in 2D arrays such as Printing
the left diagonal, right diagonal elements or Printing the elements forming the lower and
upper triangle. These problems are extremely important for your exams and placement tests!
- The left diagonal
- The right diagonal
- The lower triangle
- The upper triangle
Jump to specific sections
The left diagonal
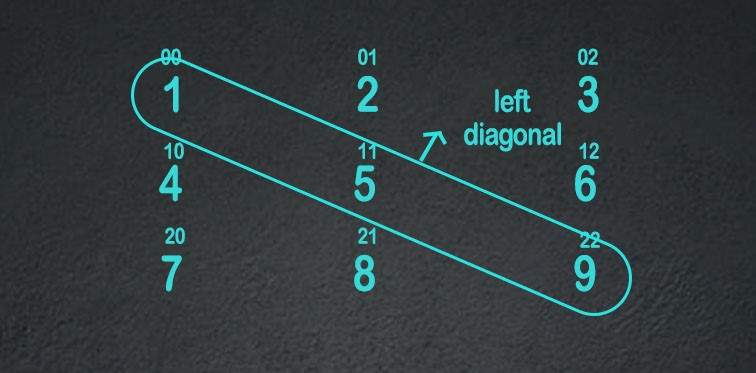
To access the elements lying on the left diagonal we write -
#include <stdio.h>
void main()
{
int a[3][3]={
{1,2,3},
{4,5,6},
{7,8,9}
};
for(int i = 0 ; i < 3 ; i++)
{
for(int j = 0 ; j < 3 ; j++)
{
if(i == j)
printf("%d",a[i][j]);
printf(" ");
}
printf("\n");
}
}
Output
1 5 9
The right diagnol
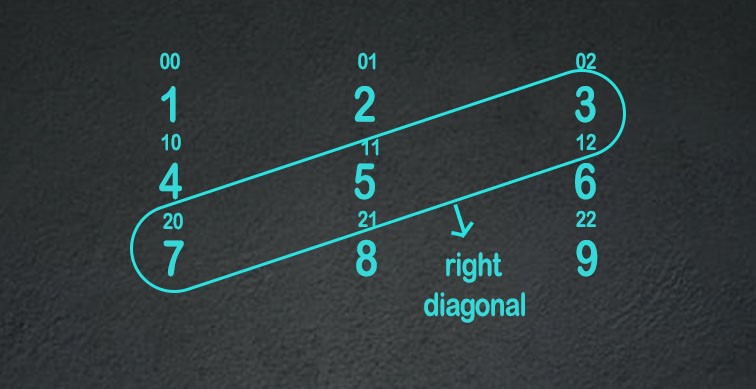
To print all the elements lying on the right diagonal we write -
#include <stdio.h>
void main()
{
int a[3][3]={
{1,2,3},
{4,5,6},
{7,8,9}
};
for(int i = 0 ; i < 3 ; i++)
{
for(int j = 0 ; j < 3 ; j++)
{
if(i+j == 2)
printf("%d",a[i][j]);
printf(" ");
}
printf("\n");
}
}
Output
3 5 7
The lower triangle
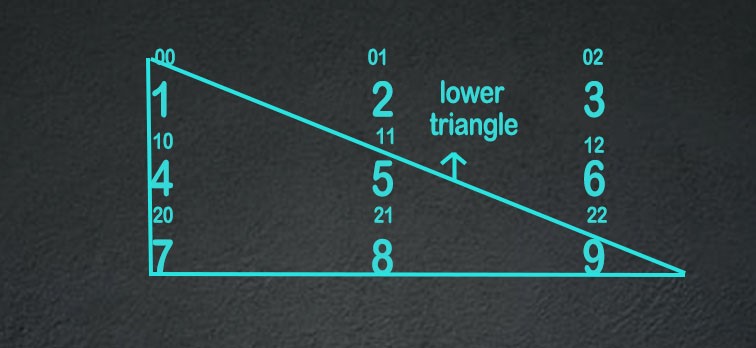
All the elements present on the left diagonal or lying below the left diagonal are included
in the lower triangle. To print all the elements lying on the lower triangle, we write -
#include <stdio.h>
void main()
{
int a[3][3]={
{1,2,3},
{4,5,6},
{7,8,9}
};
for(int i = 0 ; i < 3 ; i++)
{
for(int j = 0 ; j < 3 ; j++)
{
if(i >= j)
printf("%d",a[i][j]);
printf(" ");
}
printf("\n");
}
}
Output
1 4 5 7 8 9
The upper triangle
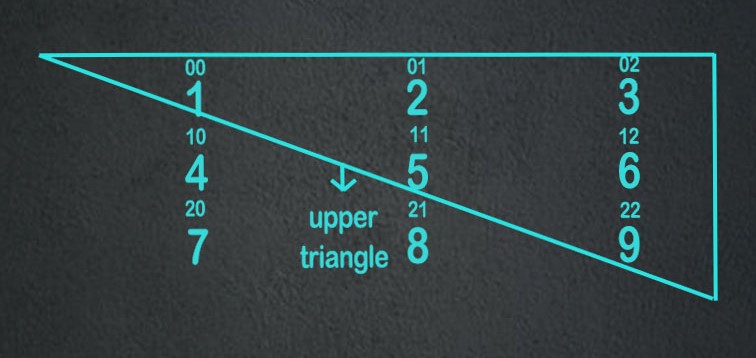
All the elements present on the right diagonal or lying below it are included
in the upper triangle. To print them, we write -
#include <stdio.h>
void main()
{
int a[3][3]={
{1,2,3},
{4,5,6},
{7,8,9}
};
for(int i = 0 ; i < 3 ; i++)
{
for(int j = 0 ; j < 3 ; j++)
{
if(i <= j)
printf("%d",a[i][j]);
else
printf(" ");
printf(" ");
}
printf("\n");
}
}
Output
1 2 3 5 6 9