Loop Control Statements in C
Introduction to HTML
How to use the Github API
The image tag, anchor tag and the button tag
Ordered and Unordered Lists in HTML
The division tag
HTML Forms
Tables in HTML
Introduction to C Programming
Introduction to Python
Varibles and Datatypes in Python
Operators in Python
Typecasting in Python
Input and Output in Python
If Else in Python
Loops in Python
Break, Continue and Pass in Python
Python practice section 1
Lists in Python
Tuple in Python
Loops in C
Loops allow us to execute a statement multiple times. Suppose that
you want to print Hello World 5 times on the screen. Using loops, you don't
have to write printf("Hello World") 5 times. Let's take a look at the below code.
#include <stdio.h>
void main()
{
for(int i = 0 ; i < 5 ; i++)
{
printf("Hello World\n");
}
}
Output
Hello World Hello World Hello World Hello World Hello World
Did you see the magic? The statement on line number 4 is called a for loop.
Using it, we were able to print Hello World 5 times without writing it 5 times.
Today, we will learn how to use loops.
- Syntax of for loop
- Working of for loop
- Example 1 - Print the table of 10
- Example 2 - Print even numbers i a given range
Jump to specific sections
What is a loop ?
Syntax of for loop
The syntax of a for loop is as follows:
for(Initialization ; Termination Condition ; Updation) { // Statement(s) }
Working of for loop
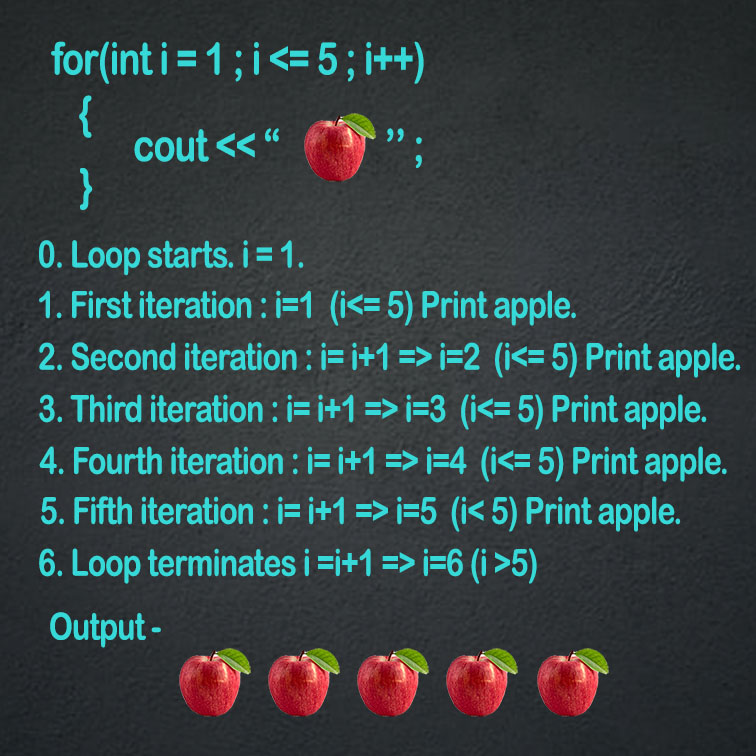
The body of a for loop is divided into three parts :
Note that if you forgot to write the updation statement or if the termination condition can never be reached(become true), then the loop keeps on running forever. Such a loop is called an infinite loop and should be avoided by taking special care while writing all the conditions.
- Initialization - This statement is executed only once when
the loop starts. It involves the declaration and initialization of a variable.
- Termination Condition - The loop must not run infinite number of times.
Hence, we specify a condition to terminate the loop. This condition is tested at
every iteration of the loop. An iteration means a cycle of the loop. If the condition is true, the loop executes the statements
inside it otherwise, the control comes out of the loop.
- Updation - It determines how to update the value of the variable declared
in the first part of the loop. We can choose any method like increment by one, decrement
by one, or increment by 2, etc. Updation is required at every iteration in order
to reach the termination condition.
Note that if you forgot to write the updation statement or if the termination condition can never be reached(become true), then the loop keeps on running forever. Such a loop is called an infinite loop and should be avoided by taking special care while writing all the conditions.
We can skip the curly brackets around the for loop if there is only one statement
inside the for loop.
Example 1 - Print the table of 10
#include <stdio.h>
void main()
{
for(int i = 1 ; i <= 10 ; i++)
{
printf("10 X %d = %d \n",i,10*i);
}
}
Output
10 X 1 = 10 10 X 2 = 20 10 X 3 = 30 10 X 4 = 40 10 X 5 = 50 10 X 6 = 60 10 X 7 = 70 10 X 8 = 80 10 X 9 = 90 10 X 10 = 100
Example 2 - Print even numbers in a given range
#include <stdio.h>
void main()
{
int a,b;
printf("Enter range : ");
scanf("%d %d",&a,&b);
for(int i = a ; i <= b ; i++)
{
if(i%2 == 0) // if i is divisible by 2, it is even.
printf("%d ",i);
}
}
Output
Enter range : 1 10 Even numbers from 1 to 10 are - 2 4 6 8 10