Loop Control Statements in C
Introduction to HTML
How to use the Github API
The image tag, anchor tag and the button tag
Ordered and Unordered Lists in HTML
The division tag
HTML Forms
Tables in HTML
Introduction to C Programming
Introduction to Python
Varibles and Datatypes in Python
Operators in Python
Typecasting in Python
Input and Output in Python
If Else in Python
Loops in Python
Break, Continue and Pass in Python
Python practice section 1
Lists in Python
Tuple in Python
1D Arrays in C
Like variables are used to store a single value. We have arrays in C that are used
to store multiple data items in one place under a common name. In other words, it is
a collection of data items of same type. For example - we can have an integer array
which stores multiple integer values or a float array to store float values. Now, we
can access the different elements in an array very easily because they are contiguous
in nature i.e. all the values in an array are stored next to each other in memory.
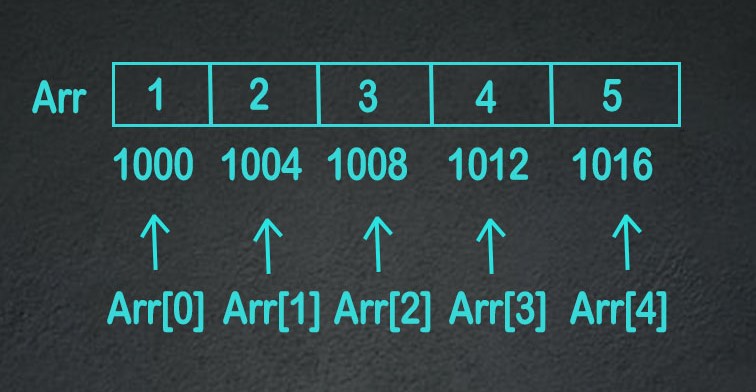
Since we know that an integer takes up 4 bytes of memory.If the starting index of the array
is 1000(say).Then the first element would be stored starting from 1000 to 1003 bytes in the memory.
The next element would be stored from 1004 to 1007 bytes and so on. In the case of a float array, each
element would take 6 bytes of memory.
Array has many applications since it can be used to store similar types of data together.
For example - to store the marks of various students in a subject, we can use an integer array,
to store the bank balances of various people in the bank, we can use a float array, etc.
Hence, it is quite a useful data structure. Enough of the theory now. Let's rapidly jump to
writing some code!
- Declaration of 1D array
- Initialization of 1D array
- Accessing the values of 1D array
- Taking 1D array as input from the user
Jump to specific sections
Declaration of 1D array
To declare a 1D array, we must specify its size in square brackets i.e. the number of elements it can store.
We also need to mention the data type of values present in it. So that proper memory
can be allocated to it.
To declare an integer array of size 5, we write -
int a[5];
To declare a float array of size 3 we write -
float f[3];
To declare a char array of size 4 we write -
char c[4];
Initialization of 1D array
To initialize an array we specify the values in curly brackets as -
int a[5]={1,2,3,4,5};
Accessing the values of 1D array
Like a book has an index that tells us the page number where each chapter is present.
In array, we also have indexes that tell where each value is located in the array. The first
value is present at the 0th index, the second value at the 1st index, and so on with the nth value
stored at the (n-1) th index.
To access a value, we specify its index in the square brackets. For example -
#include <stdio.h>
void main()
{
int a[5]={1,2,3,4,5};
printf("1st element of array = %d \n",a[0]);
printf("3rd element of array = %d \n",a[2]);
printf("5th element of array = %d \n",a[4]);
}
Output
1st element of array = 1 3rd element of array = 3 5th element of array = 5
Taking 1D array as input from the user
The first element of the array should be stored at the 0th index and so on. So, we can write
scanf("%d",&arr[0]) to store the first element and similarly for all the elements by changing
the index. Alternatively, we can use loops for the same as follows.
Consider the following example.
#include <stdio.h>
void main()
{
int n;
printf("Enter size of array : ");
scanf("%d",&n);
int arr[n];
printf("Enter the elements of array : ");
for(int i = 0 ; i < n ; i++)
{
scanf("%d",&arr[i]);
}
// Printing all the elements of the array
printf("The elements of the array are : ");
for(int i = 0 ; i < n ; i++)
{
printf("%d ",arr[i]);
}
}
Output
Enter size of array : 5 Enter the elements of array : 1 3 5 7 9 The elements of the array are : 1 3 5 7 9