Introduction to Python
Programming Cycle of Python
Varibles and Datatypes in Python
Input and Output in Python
Operators in Python
Precedence of Operators in Python
Typecasting in Python
If Else in Python
Loops in Python
Break, Continue and Pass in Python
Functions in Python
Default Arguments and Keyword Arguments in Python
Strings in python
Lists in Python
Tuple in Python
Dictionary in Python
Sets in Python
List Comprehension in Python
Unpacking Sequences in Python
Higher Order Functions in Python
Lambda Functions in Python
Sieve of Eratosthenes Algorithm in Python
Linear Search in Python
Binary Search in Python
Selection Sort in Python
Bubble Sort in Python
Insertion Sort in Python
Merge Sort in Python
Programming Cycle of Python
The Python programming life cycle is comparatively shorter and easier than the life cycles
of traditional programming languages. Python is an interpreted language i.e. it executes
the program line by line and stops at the first place it finds an error. Hence, it is easy
to debug code. There are no compile or link steps. In Python, we can simply import modules
at runtime.
Steps involved in programming cycle
- Firstly, identify the problem and start building the solution. Write the source code for implementing the solution.
- After implementing, we will test the code. The program should be kept static while testing (i.e. no changes made). Testing helps to check whether the code provides the correct solution for our problem or not.
- If we find any syntax or logical errors during testing, we will correct them by editing the source code.
- Finally, the program is ready. We can update it from time to time.
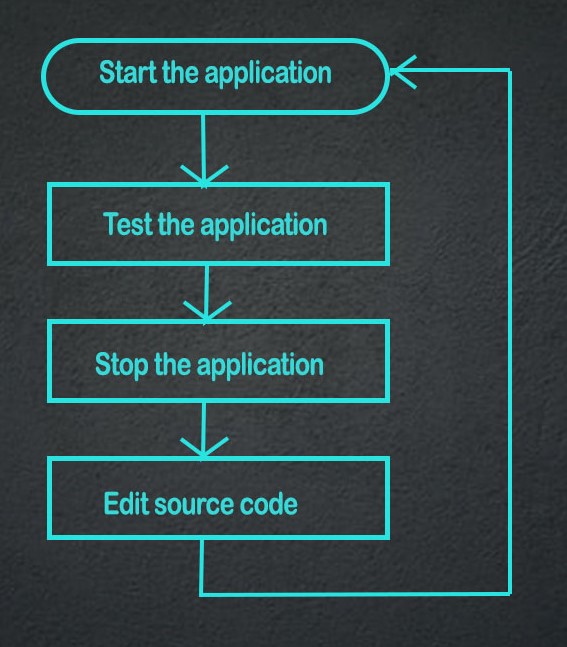